API 概述
在我们继续之前,让我们快速浏览一下 Socket.IO 提供的 API
通用 API
以下方法适用于客户端和服务器。
基本发出
正如我们在 步骤 #4 中看到的,您可以使用 socket.emit()
将任何数据发送到另一方
- 从客户端到服务器
- 从服务器到客户端
客户端
socket.emit('hello', 'world');
服务器
io.on('connection', (socket) => {
socket.on('hello', (arg) => {
console.log(arg); // 'world'
});
});
服务器
io.on('connection', (socket) => {
socket.emit('hello', 'world');
});
客户端
socket.on('hello', (arg) => {
console.log(arg); // 'world'
});
您可以发送任意数量的参数,并且所有可序列化数据结构都受支持,包括二进制对象,如 ArrayBuffer、TypedArray 或 Buffer(仅限 Node.js)
- 从客户端到服务器
- 从服务器到客户端
客户端
socket.emit('hello', 1, '2', { 3: '4', 5: Uint8Array.from([6]) });
服务器
io.on('connection', (socket) => {
socket.on('hello', (arg1, arg2, arg3) => {
console.log(arg1); // 1
console.log(arg2); // '2'
console.log(arg3); // { 3: '4', 5: <Buffer 06> }
});
});
服务器
io.on('connection', (socket) => {
socket.emit('hello', 1, '2', { 3: '4', 5: Buffer.from([6]) });
});
客户端
socket.on('hello', (arg1, arg2, arg3) => {
console.log(arg1); // 1
console.log(arg2); // '2'
console.log(arg3); // { 3: '4', 5: ArrayBuffer (1) [ 6 ] }
});
提示
不需要对对象调用 JSON.stringify()
// BAD
socket.emit('hello', JSON.stringify({ name: 'John' }));
// GOOD
socket.emit('hello', { name: 'John' });
确认
事件很棒,但在某些情况下,您可能需要更经典的请求-响应 API。在 Socket.IO 中,此功能称为“确认”。
它有两种形式
使用回调函数
您可以将回调作为 emit()
的最后一个参数添加,并且此回调将在另一方确认事件后被调用
- 从客户端到服务器
- 从服务器到客户端
客户端
socket.timeout(5000).emit('request', { foo: 'bar' }, 'baz', (err, response) => {
if (err) {
// the server did not acknowledge the event in the given delay
} else {
console.log(response.status); // 'ok'
}
});
服务器
io.on('connection', (socket) => {
socket.on('request', (arg1, arg2, callback) => {
console.log(arg1); // { foo: 'bar' }
console.log(arg2); // 'baz'
callback({
status: 'ok'
});
});
});
服务器
io.on('connection', (socket) => {
socket.timeout(5000).emit('request', { foo: 'bar' }, 'baz', (err, response) => {
if (err) {
// the client did not acknowledge the event in the given delay
} else {
console.log(response.status); // 'ok'
}
});
});
客户端
socket.on('request', (arg1, arg2, callback) => {
console.log(arg1); // { foo: 'bar' }
console.log(arg2); // 'baz'
callback({
status: 'ok'
});
});
使用 Promise
emitWithAck()
方法提供相同的功能,但返回一个 Promise,该 Promise 将在另一方确认事件后解析
- 从客户端到服务器
- 从服务器到客户端
客户端
try {
const response = await socket.timeout(5000).emitWithAck('request', { foo: 'bar' }, 'baz');
console.log(response.status); // 'ok'
} catch (e) {
// the server did not acknowledge the event in the given delay
}
服务器
io.on('connection', (socket) => {
socket.on('request', (arg1, arg2, callback) => {
console.log(arg1); // { foo: 'bar' }
console.log(arg2); // 'baz'
callback({
status: 'ok'
});
});
});
服务器
io.on('connection', async (socket) => {
try {
const response = await socket.timeout(5000).emitWithAck('request', { foo: 'bar' }, 'baz');
console.log(response.status); // 'ok'
} catch (e) {
// the client did not acknowledge the event in the given delay
}
});
客户端
socket.on('request', (arg1, arg2, callback) => {
console.log(arg1); // { foo: 'bar' }
console.log(arg2); // 'baz'
callback({
status: 'ok'
});
});
通配符监听器
通配符监听器是一个监听器,它将为任何传入事件被调用。这对于调试您的应用程序很有用
发件人
socket.emit('hello', 1, '2', { 3: '4', 5: Uint8Array.from([6]) });
接收者
socket.onAny((eventName, ...args) => {
console.log(eventName); // 'hello'
console.log(args); // [ 1, '2', { 3: '4', 5: ArrayBuffer (1) [ 6 ] } ]
});
类似地,对于传出数据包
socket.onAnyOutgoing((eventName, ...args) => {
console.log(eventName); // 'hello'
console.log(args); // [ 1, '2', { 3: '4', 5: ArrayBuffer (1) [ 6 ] } ]
});
服务器 API
广播
正如我们在 步骤 #5 中看到的,您可以使用 io.emit()
将事件广播到所有连接的客户端
io.emit('hello', 'world');
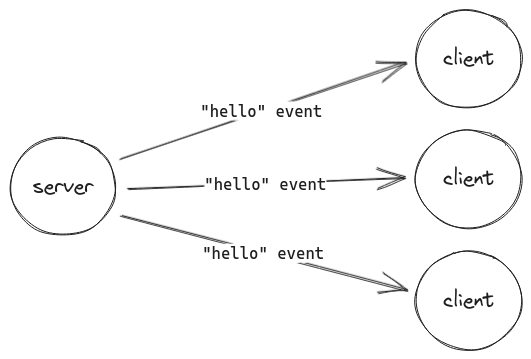
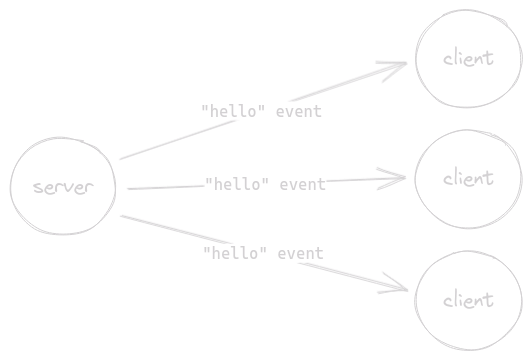
房间
在 Socket.IO 行话中,房间 是套接字可以加入和离开的任意通道。它可以用于将事件广播到连接的客户端子集
io.on('connection', (socket) => {
// join the room named 'some room'
socket.join('some room');
// broadcast to all connected clients in the room
io.to('some room').emit('hello', 'world');
// broadcast to all connected clients except those in the room
io.except('some room').emit('hello', 'world');
// leave the room
socket.leave('some room');
});
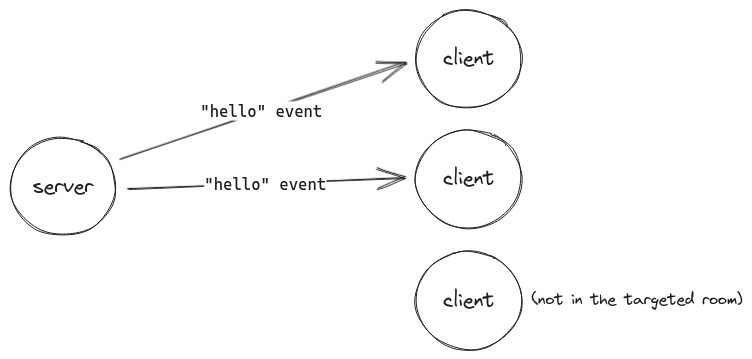
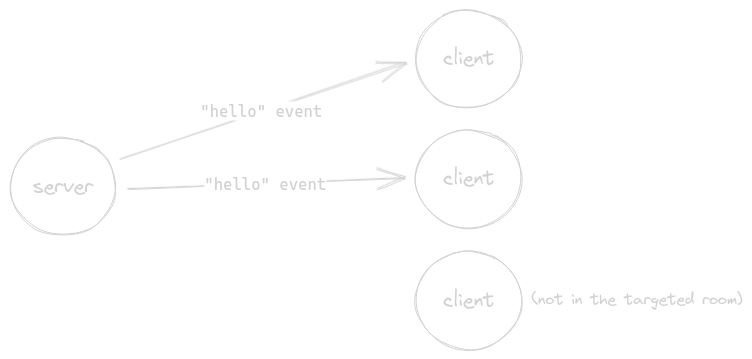